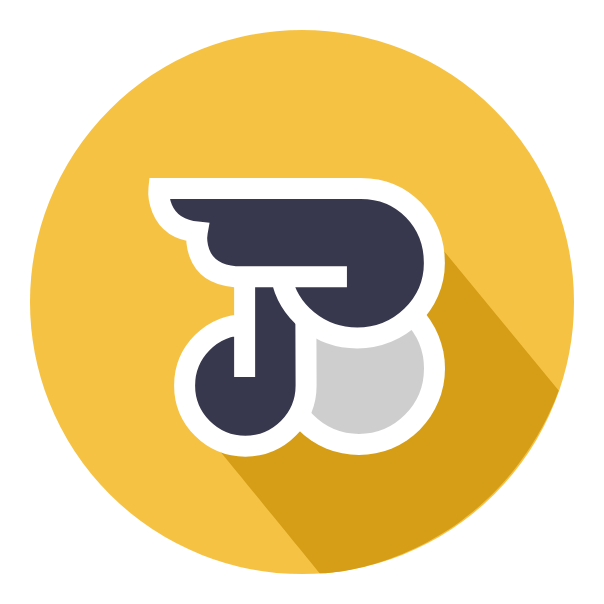
Carousel
A slideshow component for cycling through elements (images or slides of text) like a carousel.
Around component
Make sure to link to Tiny Slider css and js files in your document: vendor/tiny-slider/dist/tiny-slider.css and vendor/tiny-slider/dist/min/tiny-slider.js. Use this page as a reference.
You can alter carousel look and behaviour via modifier CSS classes and flexible data API.
Basic HTML structure:
<div class="cs-carousel">
<div class="cs-carousel-inner" data-carousel-options='{}'>
<!-- Carousel slides here -->
</div>
</div>
Modifier classes:
cs-controls-center
- Center align controls (prev/next buttons).cs-controls-left
- Left align controls (prev/next buttons).cs-controls-inside
- Position controls (prev/next buttons) absolutely on top of the carousel content (on sides). Will not work with the above positioning classes.cs-controls-onhover
- Show controls (prev/next buttons) only when user hovers over the carousel. Works only withcs-controls-inside
cs-dots-inside
- Position dots absolutely on top of the carousel content.cs-dots-light
- Switch dots skin to light version.
Data API:
data-carousel-options = '{}'
:
"mode": "carousel" | "gallery"
- With carousel everything slides to the side, while gallery uses fade animations and changes all slides at once."axis": "horizontal" | "vertical"
- The axis of the slider."items": 1
- How many items to display"nav": true/false
- Enable/disable dots control"controls": true/false
- Enable/disable prev / next arrow buttons"loop": true/false
- Enable/disable infinite loop"speed": 300
- Speed of the slide animation (in "ms")"autoplay": true/false
- Toggles the automatic change of slides"autoplayTimeout": 5000
- Timeou between transition. Value in ms | 1000ms = 1s"gutter": 0
- Space between carousel items (in px)"autoHeight": true/false
- Height of slider container changes according to each slide's height."responsive": {"0": {"items": 1}, "768": {"items": 2}, ...}
- How many items to display on each screen size. Options are not limited to number of items. You can change any option based on screen size.- For more options please visithttps://github.com/ganlanyuan/tiny-slider#options
Layer animation classes:
cs-from-top
cs-from-bottom
cs-from-left
cs-from-right
cs-scale-up
cs-scale-down
cs-fade-in
cs-delay-1
cs-delay-2
cs-delay-3
cs-delay-4
All controls: Dots + Arrows + Progress
of
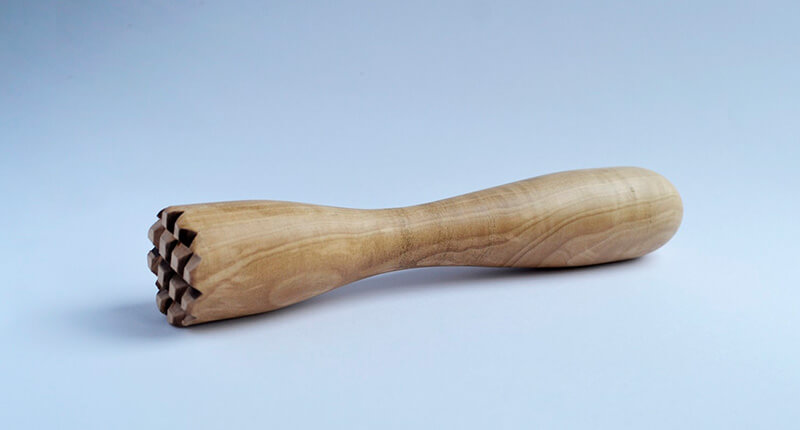
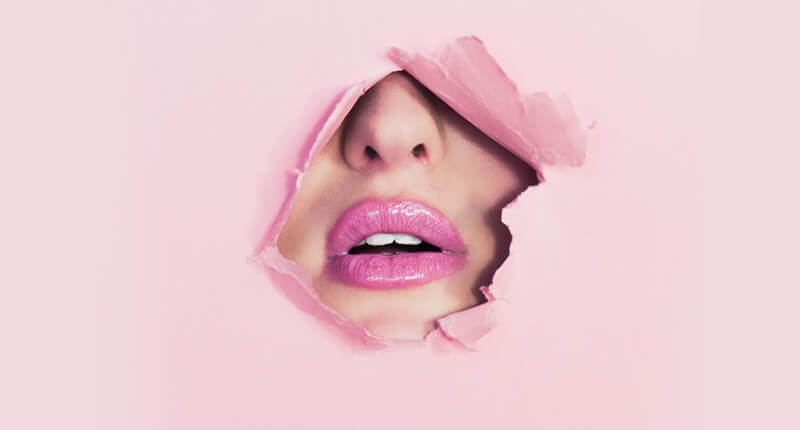
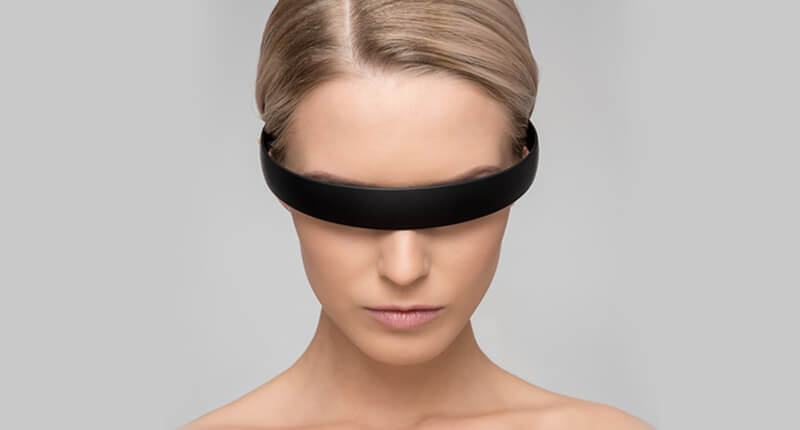
<!-- All controls: Dots + Arrows + Progress -->
<div class="cs-carousel cs-dots-inside cs-dots-light">
<div class="cs-carousel-progress ml-auto mb-3">
<div class="text-sm text-muted text-center mb-2">
<span class="cs-current-slide mr-1"></span>
of
<span class="cs-total-slides ml-1"></span>
</div>
<div class="progress">
<div class="progress-bar" role="progressbar"></div>
</div>
</div>
<div class="cs-carousel-inner" data-carousel-options='{"loop": false, "gutter": 15}'>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
</div>
</div>
// All controls: Dots + Arrows + Progress
.cs-carousel.cs-dots-inside.cs-dots-light
.cs-carousel-progress.ml-auto.mb-3
.text-sm.text-muted.text-center.mb-2
span.cs-current-slide.mr-1
| of
span.cs-total-slides.ml-1
.progress
.progress-bar(role="progressbar")
.cs-carousel-inner(data-carousel-options = '{"loop": false, "gutter": 15}')
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
Controls position: Dots outside + Arrows inside
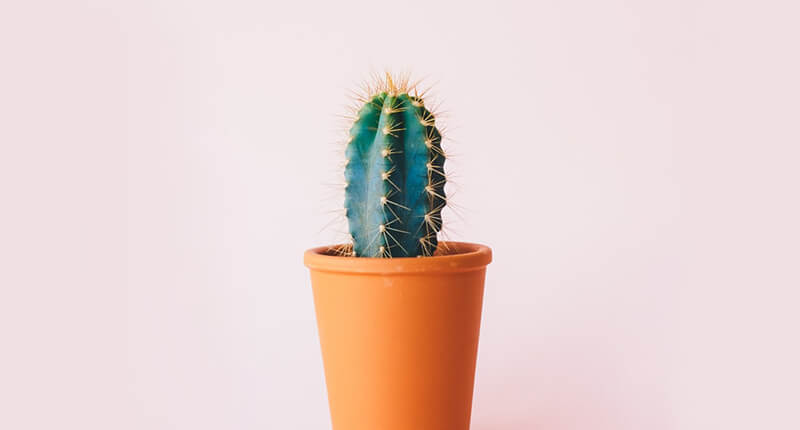
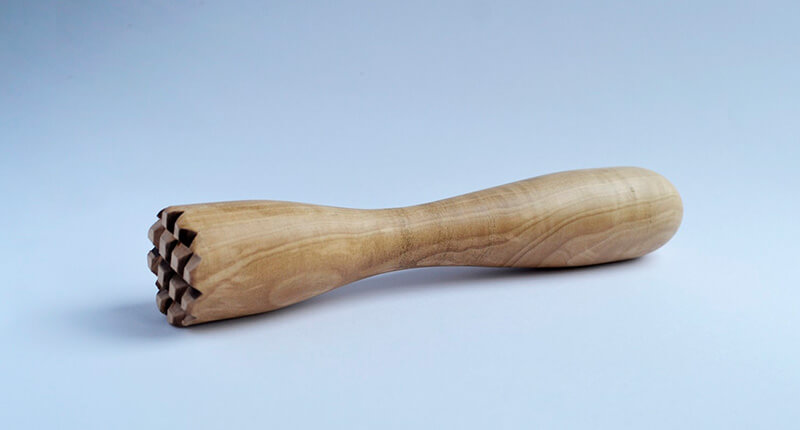
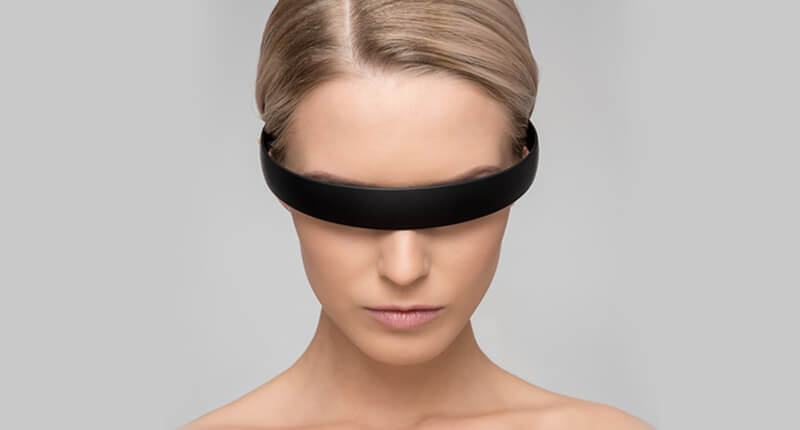
<!-- Controls position: Dots outside + Arrows inside -->
<div class="cs-carousel cs-controls-inside">
<div class="cs-carousel-inner" data-carousel-options='{"gutter": 15}'>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
</div>
</div>
// Controls position: Dots outside + Arrows inside
.cs-carousel.cs-controls-inside
.cs-carousel-inner(data-carousel-options = '{"gutter": 15}')
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
Fade transition + Layer animations
From top to bottom
From bottom to top
From left to right
From right to left
<!-- Fade transition + Layer animations -->
<div class="cs-carousel cs-controls-center">
<div class="cs-carousel-inner" data-carousel-options='{"mode": "gallery", "nav": false}'>
<div>
<div class="rounded-lg bg-faded-primary text-center py-6 px-3">
<h3 class="cs-from-top">From top to bottom</h3>
<p class="font-size-lg mb-4 pb-3 cs-from-bottom cs-delay-1">From bottom to top</p>
<button class="btn btn-primary cs-scale-down cs-delay-2" type="button">Scale down</button>
</div>
</div>
<div>
<div class="rounded-lg bg-faded-success text-center py-6 px-3">
<h3 class="cs-from-left">From left to right</h3>
<p class="font-size-lg mb-4 pb-3 cs-from-right">From right to left</p>
<button class="btn btn-success cs-scale-up cs-delay-2" type="button">Scale up</button>
</div>
</div>
</div>
</div>
// Fade transition + Layer animations
.cs-carousel.cs-controls-center
.cs-carousel-inner(data-carousel-options = '{"mode": "gallery", "nav": false}')
div
.rounded-lg.bg-faded-primary.text-center.py-6.px-3
h3.cs-from-top From top to bottom
p.font-size-lg.mb-4.pb-3.cs-from-bottom.cs-delay-1 From bottom to top
button(type="button").btn.btn-primary.cs-scale-down.cs-delay-2
| Scale down
div
.rounded-lg.bg-faded-success.text-center.py-6.px-3
h3.cs-from-left From left to right
p.font-size-lg.mb-4.pb-3.cs-from-right From right to left
button(type="button").btn.btn-success.cs-scale-up.cs-delay-2
| Scale up
Vertical carousel + Arrows visible on hover
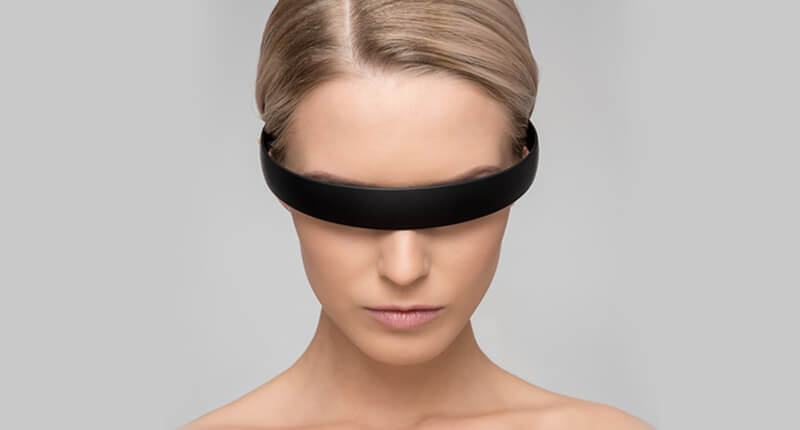
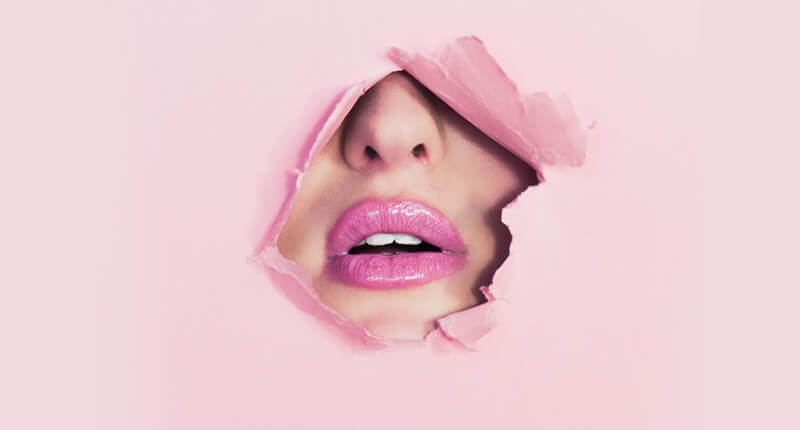
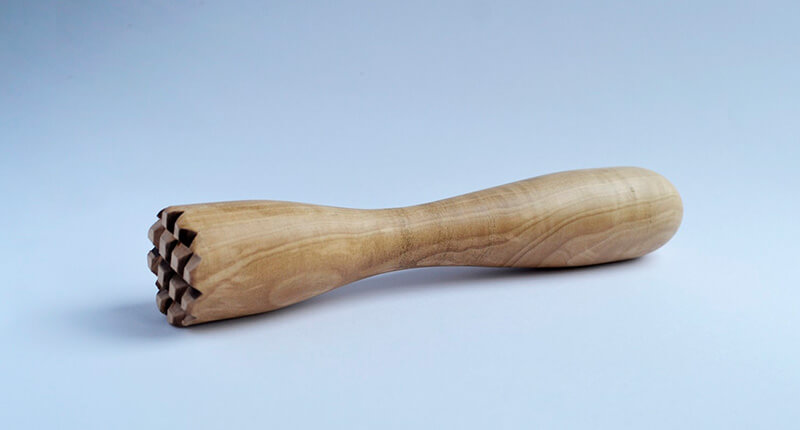
<!-- Vertical carousel + Arrows visible on hover -->
<div class="cs-carousel cs-dots-inside cs-dots-light cs-controls-inside cs-controls-onhover">
<div class="cs-carousel-inner" data-carousel-options='{"axis": "vertical", "gutter": 15}'>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded-lg" src="path-to-image" alt="Image"/>
</div>
</div>
</div>
// Vertical carousel + Arrows visible on hover
.cs-carousel.cs-dots-inside.cs-dots-light.cs-controls-inside.cs-controls-onhover
.cs-carousel-inner(data-carousel-options = '{"axis": "vertical", "gutter": 15}')
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
div
+image("path-to-image", "Image").rounded-lg
Responsive carousel with multiple items
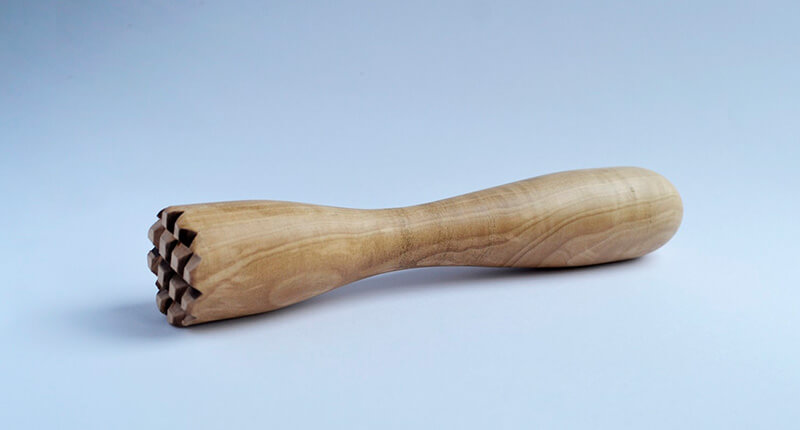
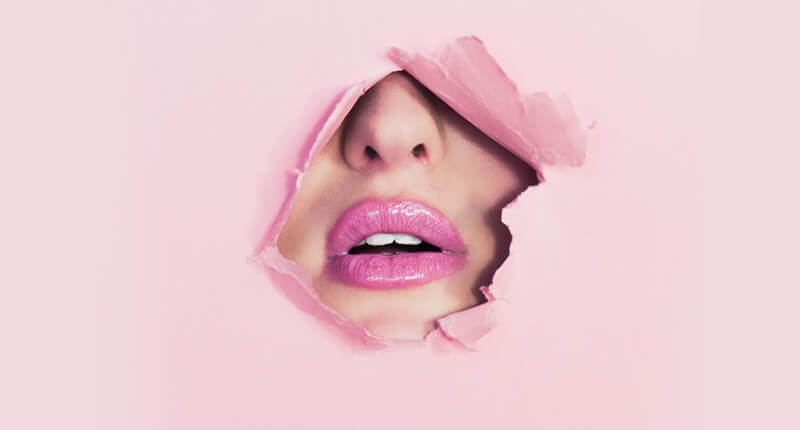
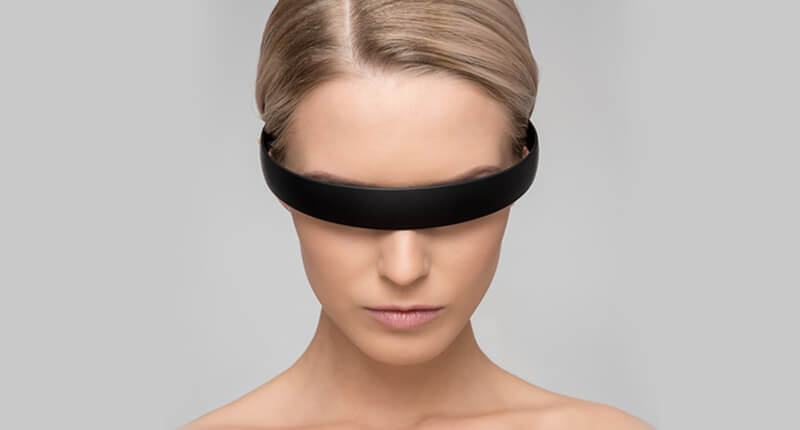
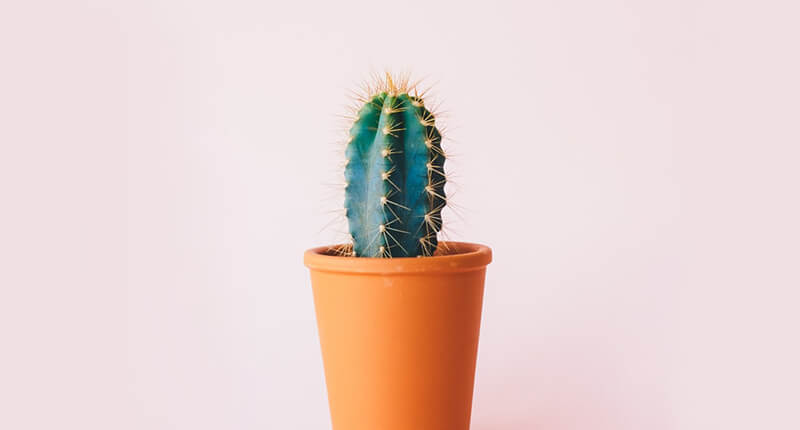
<!-- Responsive carousel with multiple items -->
<div class="cs-carousel">
<div class="cs-carousel-inner" data-carousel-options='{"items": 3, "controls": false, "responsive": {"0":{"items":1, "gutter": 16},"500":{"items":2, "gutter": 16},"768":{"items":3, "gutter": 16}, "1100":{"gutter": 24}}}'>
<div>
<img class="rounded" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded" src="path-to-image" alt="Image"/>
</div>
<div>
<img class="rounded" src="path-to-image" alt="Image"/>
</div>
</div>
</div>
// Responsive carousel with multiple items
.cs-carousel
.cs-carousel-inner(data-carousel-options = '{"items": 3, "controls": false, "responsive": {"0":{"items":1, "gutter": 16},"500":{"items":2, "gutter": 16},"768":{"items":3, "gutter": 16}, "1100":{"gutter": 24}}}')
div
+image("path-to-image", "Image").rounded
div
+image("path-to-image", "Image").rounded
div
+image("path-to-image", "Image").rounded
Default Bootstrap carousel
<!-- Default Bootstrap carousel -->
<div id="carouselExample" class="carousel slide" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carouselExample" data-slide-to="0" class="active"></li>
<li data-target="#carouselExample" data-slide-to="1"></li>
<li data-target="#carouselExample" data-slide-to="2"></li>
</ol>
<div class="carousel-inner">
<div class="carousel-item active">
<img src="path-to-image" alt="Image">
</div>
<div class="carousel-item">
<img src="path-to-image" alt="Image">
</div>
<div class="carousel-item">
<img src="path-to-image" alt="Image">
</div>
</div>
<a class="carousel-control-prev" href="#carouselExample" role="button" data-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carouselExample" role="button" data-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
// Default Bootstrap carousel
#carouselExample.carousel.slide(data-ride="carousel")
ol.carousel-indicators
li(data-target="#carouselExample", data-slide-to="0").active
li(data-target="#carouselExample", data-slide-to="1")
li(data-target="#carouselExample", data-slide-to="2")
.carousel-inner
.carousel-item.active
+image("path-to-image", "Image")
.carousel-item
+image("path-to-image", "Image")
.carousel-item
+image("path-to-image", "Image")
a(href="#carouselExample", role="button", data-slide="prev").carousel-control-prev
span(aria-hidden="true").carousel-control-prev-icon
span.sr-only Previous
a(href="#carouselExample", role="button", data-slide="next").carousel-control-next
span(aria-hidden="true").carousel-control-next-icon
span.sr-only Next